Spring Boot • Serialize Immutable Objects
Overview
This article illustrates how to serialize and write tests for immutable objects using Jackson and Lombok in Spring Boot.
Project
The maven project provided in this example used the spring initializr page https://start.spring.io to generate the initial source code.
The github repository that this article references can be found in https://github.com/kapresoft/spring-boot-serialize-immutable-objects.
For checking out and building the Spring Boot project, please refer to the README documentation on the github repository.
POM Dependencies
The spring initializr page configured the pom parent element as shown below.
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
The dependencies block will include jackson-databind and lombok. Note that developers should avoid specifying the version of the dependency so that the build will default to the spring-boot-provided version defined in the jackson-bom-<version>.pom file.
Spring boot is packaged and tested with the default lombok version in the bom file and should be beneficial to use. Developers may override the dependency version of the spring-boot-provided dependencies by specifying a different version.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Avoid declaring the version as such unless it is necessary:
<dependencies>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.5</version> <!-- <== omit this part -->
</dependency>
</dependencies>
See the full project pom.xml
Jackson
Jackson is a multi-purpose high-performance Java library used for serializing / deserializing byte streams into Java Data Transfer Objects. Developers also refer to the term “serialization” to be the same as Marshalling and Unmarshalling. The most common example is the serialization of JSON or XML byte streams into Java Objects.
Lombok
Lombok is a java library that developers use to eliminate boilerplate code such as getters and setters of a field. Lombok runs as an annotation project processor that adds generated code to your lombok-qualified classes at compile time. With the help of a plugin, most modern developer tools like IntelliJ or Visual Studio code support Lombok. In addition, the Lombok @Builder annotation is also used in this article to simplify the creation of new objects through builder factory methods.
AssertJ
AssertJ is a fluent assertion framework is an extensible component that is included with the base spring boot starter project. The framework provides readability, fluent method assertion flow, continuity, and auto-completion of methods if using an IDE. The assertion framework also encourages eliminating code duplication in your test code. There are many other benefits provided by AssertJ compared to the standard JUnit assertions.
Please visit AssertJ Document for more details.
Serialization Unit Tests
As a basic rule for developers, Unit Testing guarantees that all code meets quality standards before it’s deployed. In addition, existing Unit Tests can be used to verify a bug that may be discovered in production in the future.
Over the course of the Software Development Life Cycle (SDLC), testing your code documents the behavior, saves time and money, aids in verification and diagnostic work, and finally helps developers write better code in less time.
Writing Serialization tests for your Java Data Transport Objects are essentially cheap and easy to maintain along with utilizing an assertion framework like AssertJ.
Benefits of Immutability
Immutable objects are thread-safe and the state of the objects cannot be modified once created. Developers do not need to worry about passing in data that could be manipulated. After construction, objects can be handed-off downstream without side effects.
Serialization of Immutable Objects
Jackson provides a mechanism called Object Serialization or Deserialization where an object can be represented as JSON, XML, and others formats. This tutorial will only demonstrate serialization of JSON data to and from a Java Object.
The JSON string below can be converted or deserialized into a Java Account class object instance. In contrast, a Java Account class object instance can be converted or serialized into a JSON character stream.
Serialization
- Java Object to JSON String
Deserialization
- JSON String to Java Object
JSON Representation of an Account
For demonstration, this article uses the following JSON representation of an Account payload.
Immutable Account Class using Lombok @Value Annotation
The following example utilizes the Lombok @Value annotation to conveniently write a Java class whose instance is an immutable object.
Using the @Value annotation will produce a class with final properties or fields getter methods, a toString() and hashCode() methods. As shown on Lines 14-17 below, the ‘private final’ keywords are omitted on the property fields. Lombok will implicitly update the generated code with a ‘private final’ field. This allows developers to write less ceremonial code. How cool is that?
The java source can be viewed here for Account.java.
Unit Test for Converting Account Object to JSON String (Serialization)
For a given Account instance with values, the following unit test demonstrates the serialization of the java object into a JSON string.
@Test
void canSerializeAccount() throws JsonProcessingException {
final Account account = Account.builder()
.email("johndoe@gmail.com").username("johndoe")
.firstName("John").lastName("Doe")
.build();
final String jsonText = mapper.writeValueAsString(account);
final String expectedJSON = "{\"username\":\"johndoe\",\"email\":\"johndoe@gmail.com\",\"firstName\":\"John\",\"lastName\":\"Doe\"}";
assertThat(jsonText).as("Expected Account JSON")
.isEqualTo(expectedJSON);
}
The java source can be viewed here for AccountSerializationTest.java.
Unit Test for Converting JSON String to Account Object (Deserialization)
Given the following JSON string content depicted in section 3.1, the following unit test demonstrates the JSON deserialization into an Account object.
@Test
void canDeserializeAccountJSON() throws JsonProcessingException {
// See section 3.1 for the JSON string
final Account account = mapper.readValue(JSON, Account.class);
assertThat(account).as("Account")
.isNotNull();
assertThat(account.getUsername()).as("Username")
.isEqualTo("kapre");
assertThat(account.getEmail()).as("Email")
.isEqualTo("kapre@kapresoft.com");
}
The java source can be viewed here for AccountSerializationTest.java.
Immutable Account Class without using Lombok @Value Annotation
The following example is an alternate implementation of an Account class in the previous section that doesn’t use @Value, but instead uses @Getter and specifies explicitly additional annotations to represent a java immutable object.
In the previous section the @Value represented an annotation provided by Lombok to represent a series of configuration that will generate code during compile time representing an immutable class. Developers can choose not to use @Value for design reasons and doing so will require adding or unwrapping the other required Lombok annotations.
The following java code is equivalent to the previous code using @Value with the addition of @AllArgsConstructor, @ToString, @EqualsAndHashCode, and @Getter annotations.
As you can see from this implementation that the first Account implementation in 3.2 is less convoluted with Lombok Annotations.
Either use of Lombok @Value or the explicit @Getter shown on sections 3.2 and 3.3 should not affect testability. The test written for 3.2 implementation should pass for 3.3 and vice versa.
The java source can be viewed here for AccountWithoutUsingValue.java.
Unit Test for Converting AccountWithoutUsingValue Object to JSON String (Serialization)
For a given AccountWithoutUsingValue instance with values, the following unit test demonstrates the serialization of the java object into a JSON string.
@Test
void canSerializeAccount_WithoutUsingValue() throws JsonProcessingException {
final AccountWithoutUsingValue account = AccountWithoutUsingValue.builder()
.email("johndoe@gmail.com").username("johndoe")
.firstName("John").lastName("Doe")
.build();
final String jsonText = mapper.writeValueAsString(account);
final String expectedJSON = "{\"username\":\"johndoe\",\"email\":\"johndoe@gmail.com\",\"firstName\":\"John\",\"lastName\":\"Doe\"}";
assertThat(jsonText).as("Expected Account JSON")
.isEqualTo(expectedJSON);
}
The java source can be viewed here for AccountSerializationTest.java.
Unit Test for Converting JSON String to AccountWithoutUsingValue Object (Deserialization)
Given the following JSON string content depicted in section 3.1, the following unit test demonstrates the JSON deserialization into an AccountWithoutUsingValue object.
@Test
void canDeserializeAccountJSON_WithoutUsingValue() throws JsonProcessingException {
// See section 3.1 for the JSON string
final AccountWithoutUsingValue account = mapper.readValue(JSON, AccountWithoutUsingValue.class);
assertThat(account).as("Account")
.isNotNull();
assertThat(account.getUsername()).as("Username")
.isEqualTo("kapre");
assertThat(account.getEmail()).as("Email")
.isEqualTo("kapre@kapresoft.com");
}
The java source can be viewed here for AccountSerializationTest.java.
Immutability and Inheritance
Immutability and Lombok also works well with a hierarchical or parent-child object structure. HierarchicalAccount class which extends from a BaseAccount class is implemented in Jackson as shown in the next two sections.
BaseAccount Class
The BaseAccount is an abstract class and must declare a @Getter annotation for the property getter methods.
The java source can be viewed here for BaseAccount.java
HierarchicalAccount Class
For this parent-child hierarchical structure, the figure below shows that HierarchicalAccount class extends from the parent BaseAccount class.
Figure 3.4.2.1. HierarchicalAccount Class Diagram
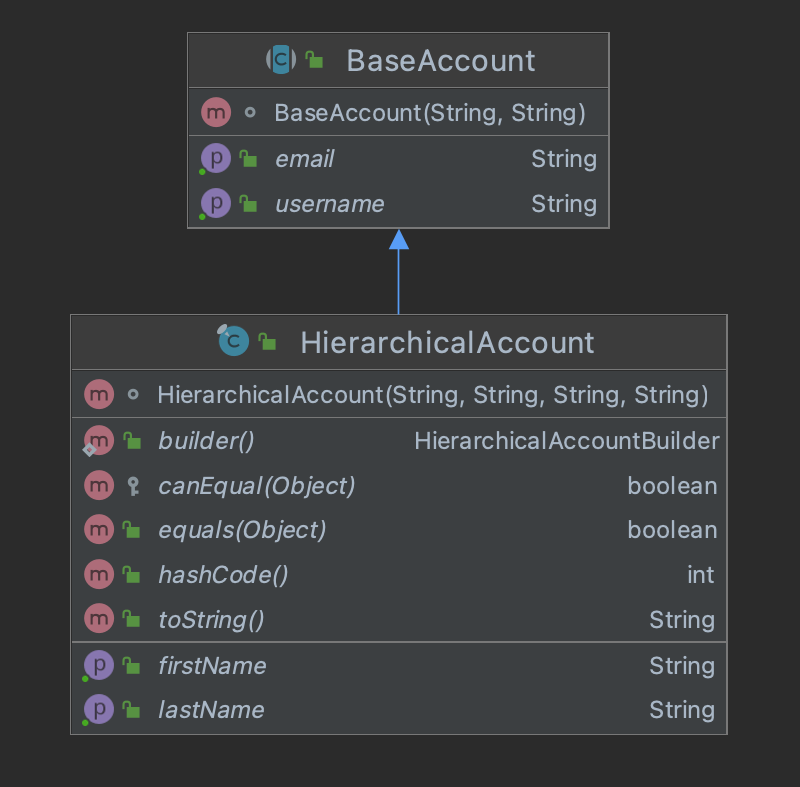
Lombok updates to handle a hierarchical structure:
- Annotate the type with @EqualsAndHashCode(callSuper = true)
- Hints Lombok code generation to take into account the parent class hashCode() method when generating its own hashCode() method.
- Call the parent super(username: String, email: String) method
- Initializes the parent class constructor accordingly.
The java source can be viewed here for HierarchicalAccount.java
Unit Test for Converting HierarchicalAccount Object to JSON String (Serialization)
For a given HierarchicalAccount instance with values, the following unit test demonstrates the serialization of the java object into a JSON string.
@Test
void canSerializeHierarchicalAccount() throws JsonProcessingException {
final HierarchicalAccount account = HierarchicalAccount.builder()
.email("johndoe@gmail.com").username("johndoe")
.firstName("John").lastName("Doe")
.build();
final String jsonText = mapper.writeValueAsString(account);
final String expectedJSON = "{\"username\":\"johndoe\",\"email\":\"johndoe@gmail.com\",\"firstName\":\"John\",\"lastName\":\"Doe\"}";
assertThat(jsonText).as("Expected Account JSON")
.isEqualTo(expectedJSON);
}
The java source can be viewed here for HierarchicalAccountSerializationTest.java.
Unit Test for Converting JSON String to HierarchicalAccount Object (Deserialization)
Given the following JSON string content depicted in section 3.1, the following unit test demonstrates the JSON deserialization into an HierarchicalAccount object.
@Test
void canDeserializeHierarchicalAccount() throws JsonProcessingException {
// See section 3.1 for the JSON string
final HierarchicalAccount account = mapper.readValue(JSON, HierarchicalAccount.class);
assertThat(account).as("Account")
.isNotNull();
assertThat(account.getUsername()).as("Username")
.isEqualTo("kapre");
assertThat(account.getEmail()).as("Email")
.isEqualTo("kapre@kapresoft.com");
}
The java source can be viewed here for HierarchicalAccountSerializationTest.java.
Summary
This article in summary discussed the following points:
- Discussed usage and simplicity of using Lombok @Value and @Getter for Immutable Objects.
- Demonstrated the use of Jackson and Lombok on hierarchical or parent-child class structures.
- Speed, Maintainability and the cleanliness of code when using Lombok Annotations.
- Provided Unit Tests to verify serialization of java objects and deserialization into JSON strings.
Spring • Intro to WebTestClient
Post Date: 10 Jan 2024
In the ever-evolving landscape of web application development, the Spring Framework stands out as a robust, versatile platform. Among its myriad tools and features, WebTestClient emerges as a pivotal component, especially in the realm of testing. This introductory article will navigate through the basics of WebTestClient, unraveling its role in enhancing the testing capabilities of Spring-based web applications.
Spring • Intro To Null Safety
Post Date: 09 Jan 2024
The Spring Framework brings a pivotal enhancement to Java’s capabilities with its introduction of null safety annotations. This article aims to unravel how these annotations bridge the gap created by Java’s limited ability to express null safety through its type system.
Spring • Intro To Bean Post Processors
Post Date: 08 Jan 2024
The Spring Framework, a cornerstone for developing modern Java applications, is renowned for its comprehensive capabilities in managing and enhancing Java beans. A pivotal component in this toolkit is the BeanPostProcessors. These elements are instrumental in tailoring the bean creation and lifecycle management process, offering developers granular control over bean behavior. This article delves deep into the realm of BeanPostProcessors, unraveling their functional dynamics, significance, and methodologies for effective utilization.
Spring • Intro to Java-based Configuration
Post Date: 06 Jan 2024
In this article, we delve into the transformative world of Java-based configuration in Spring Framework. We begin by exploring the evolution from traditional XML configurations to the more dynamic Java-based approach, highlighting the advantages and flexibility it brings to modern software development. This introduction sets the stage for a comprehensive understanding of Java-based configuration in Spring, offering insights into why it has become a preferred method for developers worldwide.
Autowiring With Factory Beans in Spring
Post Date: 06 Jan 2024
The Spring Framework, a cornerstone in the world of Java application development, has revolutionized the way developers manage dependencies. At the heart of this transformation is the concept of Autowiring, a powerful feature that automates the process of connecting objects together. Autowiring in Spring eliminates the need for manual wiring in XML configuration files, instead relying on the framework’s ability to intuitively ‘guess’ and inject dependencies where needed. This intuitive approach not only simplifies the code but also enhances its modularity and readability, making Spring-based applications more maintainable and scalable.
Spring • Web Mvc Functional Endpoints
Post Date: 05 Jan 2024
In the dynamic landscape of web development, the Spring Framework has emerged as a cornerstone for building robust and scalable web applications. At the heart of this framework lies Spring Web MVC, a powerful module known for its flexibility and ease of use. This article aims to shed light on a particularly intriguing aspect of Spring Web MVC: WebMvc.fn, an approach that represents a more functional style of defining web endpoints.
Spring • Revolutionize the Power of Strongly Typed @Qualifiers.
Post Date: 05 Jan 2024
The Spring Framework, renowned for its comprehensive infrastructure support for developing robust Java applications, empowers developers with various tools and annotations to streamline the process. One such powerful annotation is @Qualifier, which refines the autowiring process in Spring applications. This article delves into the basic usage of @Qualifier in conjunction with Spring’s autowiring feature and then explores a more advanced technique: creating a strongly-typed qualifier through custom annotation. It focuses on how these methods enhance precision in dependency injection, using Spring Boot as the demonstration platform.
Spring • Intro to @SessionScope
Post Date: 04 Jan 2024
In the world of Spring Framework, understanding session scope is crucial for efficient web application development. This article serves as an introduction to the concept of session scope in Spring and sheds light on its significance in managing user sessions within web applications. We’ll delve into the fundamentals and explore why it plays a pivotal role in creating responsive and user-centric web experiences.
Spring • Intro To Prototype Scope
Post Date: 04 Jan 2024
In this article, we’ll dive into one of the less explored yet highly valuable concepts in the Spring Framework - the Prototype scope. While many developers are familiar with the more common scopes like @Singleton and @Request, understanding the nuances of Prototype can give you more control over the lifecycle of your Spring beans. We’ll explore what Prototype scope is, when and why you should use it, and how it differs from other scopes.
Spring • Intro to @ApplicationScope
Post Date: 04 Jan 2024
The Spring Framework is a foundational element in the realm of enterprise application development, known for its powerful and flexible structures that enable developers to build robust applications. Central to effectively utilizing the Spring Framework is a thorough understanding of its various scopes, with a special emphasis on @ApplicationScope. This scope is crucial for optimizing bean management and ensuring efficient application performance.
Getting Started with Spring Framework
Post Date: 03 Jan 2024
The Spring Framework stands as a cornerstone in the world of Java application development, representing a paradigm shift in how developers approach Java Enterprise Edition (Java EE). With its robust programming and configuration model, Spring has streamlined the complexities traditionally associated with Java EE. This article aims to illuminate the core aspects of the Spring Framework, shedding light on its pivotal role in enhancing and simplifying Java EE development. Through an exploration of its features and capabilities, we unveil how Spring not only elevates the development process but also reshapes the landscape of enterprise Java applications.
Transform Your Data: Advanced List Conversion Techniques in Spring
Post Date: 02 Jan 2024
The ConversionService of the Spring Framework plays a crucial role in simplifying data conversion tasks, particularly for converting lists from one type to another. This article zeroes in on understanding and leveraging the Spring Conversion Service specifically for list conversions, an essential skill for effective and accurate coding in Spring applications.
Mastering Spring's Scopes: A Beginner's Guide to Request Scope and Beyond
Post Date: 24 Dec 2023
Spring Framework, a powerful tool in the Java ecosystem, offers a variety of scopes for bean management, critical for efficient application development. Among these, Request Scope is particularly important for web applications. This article dives deep into the nuances of Request Scope, especially for beginners, unraveling its concept and comparing it with the Prototype Scope.
Decoding AOP: A Comprehensive Comparison of Spring AOP and AspectJ
Post Date: 24 Dec 2023
In this comprehensive comparison, we dive into the intricate world of Aspect-Oriented Programming (AOP) with a focus on two prominent players: Spring AOP and AspectJ. Understanding the distinction between these two technologies is crucial for software developers and architects looking to implement AOP in their applications.
Spring • Overcoming AOP Internal Call Limitation
Post Date: 14 Dec 2023
Aspect-Oriented Programming (AOP) in Spring offers a powerful way to encapsulate cross-cutting concerns, like logging, security, or transaction management, separate from the main business logic. However, it’s not without its limitations, one of which becomes evident in the context of internal method calls.
Spring • Custom Annotations & AnnotationUtils
Post Date: 06 Dec 2023
Spring, a powerhouse in the Java ecosystem, is renowned for simplifying the development process of stand-alone, production-grade Spring-based applications. At its core, Spring leverages annotations, a form of metadata that provides data about a program but isn’t part of the program itself. These annotations are pivotal in reducing boilerplate code, making your codebase cleaner and more maintainable.
Spring • Custom Annotations & AspectJ In Action
Post Date: 06 Dec 2023
In this article, we delve into the dynamic world of Spring Framework, focusing on the power of custom annotations combined with AspectJ. We’ll explore how these technologies work together to enhance the capabilities of Spring applications. For those already versed in Spring and the art of crafting custom annotations in Java, this piece offers a deeper understanding of integrating AspectJ for more robust and efficient software design.
Mastering Testing with @MockBean in Spring Boot
Post Date: 01 Dec 2023
In the realm of Java application development, the @MockBean annotation in Spring Boot is pivotal for effective testing. Part of the org.springframework.boot.test.mock.mockito package, it facilitates the creation and injection of Mockito mock instances into the application context. Whether applied at the class level or on fields within configuration or test classes, @MockBean simplifies the process of replacing or adding beans in the Spring context.
Spring Boot MockMVC Best Practices
Post Date: 16 Nov 2023
Spring MockMVC stands as a pivotal component in the Spring framework, offering developers a robust testing framework for web applications. In this article, we delve into the nuanced aspects of MockMVC testing, addressing key questions such as whether MockMVC is a unit or integration test tool, its best practices in Spring Boot, and how it compares and contrasts with Mockito.
Spring Boot • Logging with Logback
Post Date: 19 Oct 2023
When it comes to developing robust applications using the Spring framework, one of the key aspects that developers need to focus on is logging. Logging in Spring Boot is a crucial component that allows you to keep track of the behavior and state of your application.
Spring • DevOps Best Practices with Spring Profiles
Post Date: 19 Oct 2023
The integration of Spring with DevOps practices is integral to modern application development. This guide will provide a deep dive into managing Spring profiles efficiently within machine images like Docker, including essential security-specific configurations for production Spring profiles and the handling of AWS resources and secret keys.
Spring Boot • Environment Specific Profiles
Post Date: 19 Oct 2023
When building a Spring Boot application, it’s essential to have different configurations for various environments like development (dev), testing (test), integration, and production (prod). This flexibility ensures that the application runs optimally in each environment.
Spring WebFlux/Reactive • Frequently Asked Questions
Post Date: 17 Oct 2023
In the evolving landscape of web development, reactive programming has emerged as a game-changer, offering solutions to modern high-concurrency, low-latency demands. At the forefront of this shift in the Java ecosystem is Spring WebFlux, an innovative framework that champions the reactive paradigm.
Spring Validation • Configuring Global Datetime Format
Post Date: 17 Oct 2023
In the world of Java development, ensuring proper data validation and formatting is crucial. One key aspect of this is configuring a global date and time format. In this article, we will delve into how to achieve this using the Spring Framework, specifically focusing on Java Bean Validation.
Spring Java Bean Validation
Post Date: 17 Oct 2023
The Spring Framework, renowned for its versatility and efficiency, plays a pivotal role in offering comprehensive support for the Java Bean Validation API. Let’s embark on an exploration into the world of Bean Validation with Spring.
Spring 5 • Getting Started With Validation
Post Date: 17 Oct 2023
Validation is an essential aspect of any Spring Boot application. Employing rigorous validation logic ensures that your application remains secure and efficient. This article discusses various ways to integrate Bean Validation into your Spring Boot application within the Java ecosystem. We’ll also explore how to avoid common pitfalls and improve your validation processes.
Spring 6 • What's New & Migration Guide
Post Date: 16 Oct 2023
The Spring Framework’s legacy in the Java ecosystem is undeniable. Recognized for its powerful architecture, versatility, and constant growth, Spring remains at the forefront of Java development. The release of Spring Framework 6.x heralds a new era, with enhanced features and revisions that cater to the modern developer’s needs.
Spring UriComponentsBuilder Best Practices
Post Date: 16 Oct 2023
The Spring Framework offers an array of robust tools for web developers, and one such utility is the UriComponentsBuilder. This tool provides an elegant and fluent API for building and manipulating URIs. This article offers a deep dive into various methods and applications of UriComponentsBuilder, backed by practical examples.
Spring Field Formatting
Post Date: 02 Oct 2023
Spring Field Formatting is a pivotal component of the Spring Framework, allowing seamless data conversion and rendering across various contexts, particularly in client environments. This guide provides an in-depth look into the mechanics, interfaces, and practical implementations of Spring Field Formatting, elucidating its significance in modern web and desktop applications.
Spring Validator • Resolving Error Codes
Post Date: 01 Oct 2023
Data validation is paramount for web applications, ensuring user input aligns with application expectations. Within the Spring ecosystem, validation and error message translation are critical components, enhancing user experience.
Spring Validator Interface
Post Date: 01 Oct 2023
Spring offers a robust framework for application developers, with one of its standout features being data validation. Validation is essential for ensuring the accuracy, reliability, and security of user input. In this guide, we’ll delve deep into Spring’s Validator interface, understand its significance in the context of web applications, and explore how to implement it effectively.
Spring Type Conversion
Post Date: 01 Oct 2023
Spring provides a robust type conversion system through its core.convert package, offering a versatile mechanism for converting data types within your applications. This system leverages an SPI (Service Provider Interface) for implementing type conversion logic and a user-friendly API for executing these conversions during runtime.
Spring Framework Expression Language
Post Date: 01 Oct 2023
Spring, the ever-evolving and popular framework for Java development, offers a myriad of functionalities. Among these, the Spring Expression Language (SpEL) stands out as a notable feature for its capability to manipulate and query object graphs dynamically. In this comprehensive guide, we unravel the intricacies of SpEL, shedding light on its operators, syntax, and application.
Spring Framework Annotations
Post Date: 01 Oct 2023
Spring Framework has solidified its place in the realm of Java-based enterprise applications. Its annotations simplify the coding process, enabling developers to focus on the business logic. This article delves into the core annotations in the Spring Framework, shedding light on their purposes and usage. Through this comprehensive guide, we aim to provide clarity and depth on these annotations.
Spring Controller vs RestController
Post Date: 01 Oct 2023
The Spring MVC framework stands out as one of the most robust and versatile frameworks in the realm of Java web development. At the heart of its dynamism are two key annotations: @Controller and @RestController. These annotations not only define the structure but also dictate the behavior of web applications. This exploration aims to provide a deeper understanding of these annotations, their respective functionalities, and when to optimally use them.
Spring Boot Conditional Annotations
Post Date: 01 Oct 2023
The world of Java programming, notably within the Spring Framework, constantly evolves, offering developers powerful tools and techniques to streamline application building. One such tool that stands out is the @Conditional annotation. This robust tool in Spring Boot is an absolute game-changer, offering a range of built-in annotations that allow developers to control configurations based on multiple criteria.
Spring Bean Manipulation and the BeanWrapper
Post Date: 01 Oct 2023
In the realm of Java-based applications, the Spring Framework is renowned for providing powerful tools to manipulate and manage bean objects. Central to this process is the BeanWrapper. This article delves into the essence of Bean Manipulation, shedding light on the BeanWrapper, and the various tools provided by the Spring Framework and java.beans package.
Managing AWS CloudFront Using Spring Shell
Post Date: 26 May 2023
This article explores an efficient approach to deploying static pages in CloudFront while leveraging the content delivery capabilities of AWS S3 and the convenience of Spring Shell Command-Line Interface (CLI) using the AWS SDK for Java.
Spring Framework Events
Post Date: 17 May 2023
Spring Framework provides a powerful event handling mechanism that allows components within an application context to communicate and respond to events. This mechanism is based on the Observer design pattern and is implemented using the ApplicationEvent class and the ApplicationListener interface.
Spring Bean Scopes
Post Date: 16 May 2023
Understanding and Utilizing Bean Scopes in the Spring Framework
In this article, we will delve into the concept of bean scopes in Spring Framework. Understanding and effectively utilizing bean scopes is essential for controlling the lifecycle and behavior of your beans, allowing you to enhance the flexibility and power of your Spring applications.
Spring 6 Error Handling Best Practices
Post Date: 15 May 2023
Error handling and exception design are integral components of developing Spring RESTful APIs, ensuring the application’s reliability, stability, and user experience. These practices enable developers to effectively address unexpected scenarios, such as invalid requests, database errors, or service failures, by providing graceful error responses.
Spring Boot, Jackson, and Lombok Best Practices
Post Date: 12 Apr 2023
This article discusses the recommended practices for using Jackson and Lombok in conjunction with Spring Boot, a popular framework for building enterprise-level Java applications.
Encrypting Properties File Values with Jasypt
Post Date: 28 Mar 2023
Property files are text resources in your standard web application that contains key-value information. There may come a time when information should not be stored in plain sight. This article will demonstrate how to encrypt properties file values using Jasypt encryption module. Jasypt is freely available and comes with Spring Framework integration.
Spring Boot Profiles & AWS Lambda: Deployment Guide
Post Date: 20 Oct 2019
In this article, we will explore how to leverage the Spring Boot Profiles feature in an AWS Lambda Compute environment to configure and activate specific settings for each environment, such as development, testing, integration, and production.
AWS Lambda with Spring Boot: A Comprehensive Guide
Post Date: 28 Apr 2019
This article explores the benefits of using Spring Boot with AWS Lambda, a powerful serverless compute service that enables developers to run code without worrying about server management. By integrating with the AWS cloud, AWS Lambda can respond to a variety of AWS events, such as S3, Messaging Gateways, API Gateway, and other generic AWS Resource events, providing an efficient and scalable solution for your application needs.
Secure SMTP with Spring JavaMailSender
Post Date: 15 May 2016
This article discusses the use of Java Mail in the context of migrating email services to Google Apps For Your Domain. The author shares their experience with using the free service and encountered a problem with using the secure SMTP protocol to send emails programmatically through their old email account with the Spring JavaMailSender.