Java • Are Static Classes Things Of The Past?
Overview
Static classes have been a staple in the programming world for decades. Traditionally, a static class is one where all members and functions are static, meaning they belong to the class itself rather than any specific instance of the class. This makes static classes an efficient tool for grouping related functions and data that do not require object instantiation to be accessed. For instance, in a language like C#, the Math class is a classic example, providing mathematical functions like Math.Sqrt() that can be called without creating an instance of the Math class.
Static classes are often used for their simplicity and ease of access. They serve as a home for utility functions and constants that are relevant throughout the application, without the overhead of creating objects. This approach simplifies code and improves performance in scenarios where state preservation across instances is unnecessary.
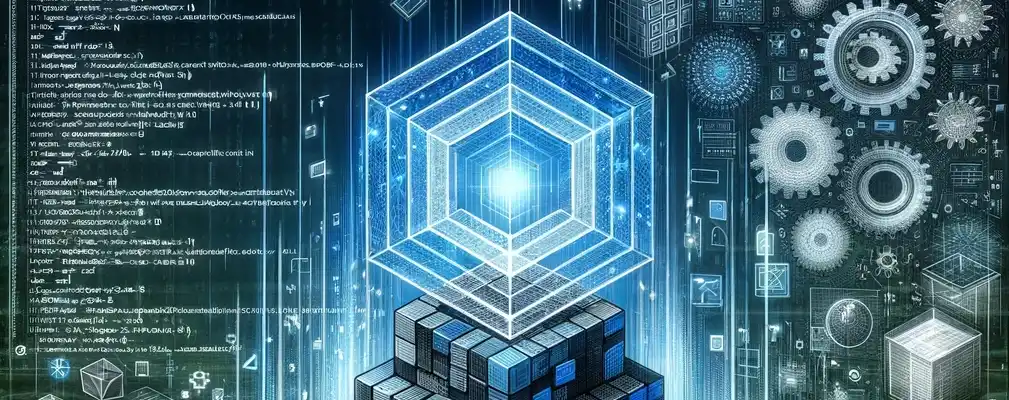
The Current Debate on Static Classes
In the ever-evolving landscape of software development, the role and relevance of static classes have come under scrutiny. As programming paradigms shift towards more flexible, object-oriented, and modular designs, the static class, with its rigid structure and global state management, seems increasingly out of place.
The debate intensifies particularly in contexts where software design principles, like SOLID and design patterns, emphasize decoupling, testability, and maintainability. Critics argue that static classes can lead to code that is harder to test and maintain due to their global state and tight coupling. They also point out that static classes do not fit well into paradigms like dependency injection, which are central to modern application frameworks.
Supporters of static classes, however, highlight their continued usefulness in certain contexts. They point out scenarios where static classes offer a clear, straightforward solution without the added complexity of object instantiation. For instance, in a Java application, utility classes like java.util.Collections provide static methods to operate on collections, which are simpler and more efficient for certain operations than creating separate utility objects.
As we delve deeper into this article, we will explore how static classes are being adapted and used in contemporary programming, particularly in Java, and weigh their benefits against the emerging paradigms and practices in software development.
The Evolution of Static Classes
The concept of static classes has its roots in the early days of procedural programming. Initially, programming languages like C emphasized a structured approach, where the focus was on procedures or functions rather than objects. In this context, static functions and variables played a crucial role. They provided a way to maintain state and execute logic without the need for instantiating objects. For instance, a static counter variable in a function could be used to track the number of times the function was called.
As programming languages evolved, static constructs were integrated into their designs to facilitate global access and utility operations. In languages like C++, static members within classes allowed for shared data and methods relevant to the class as a whole, rather than individual instances. This was especially useful for utility operations and constants.
Transition from Structured to Object-Oriented Programming (OOP)
The advent of object-oriented programming (OOP) marked a significant shift in the way developers approached software design. OOP languages like Java and C++ brought the concept of objects to the forefront, emphasizing encapsulation, inheritance, and polymorphism. This paradigm shift had a profound impact on the use of static classes.
In OOP, the emphasis is on creating objects as instances of classes, each with their own state and behavior. This contrasts with the static approach, where methods and variables exist independently of object instances. While static classes still held their ground for utility purposes, the focus shifted towards designing more flexible, modular, and maintainable systems using object instances.
C Example
In C, a structured programming language, functions and variables are typically defined globally or within modules. For instance, a function to calculate the area of a circle might be defined as:
#include <stdio.h>
#define PI 3.14159
double circleArea(double radius) {
return PI * radius * radius;
}
int main() {
printf("Area: %f\n", circleArea(5.0));
return 0;
}
Here, circleArea is a global function, accessible throughout the program.
C++ Example
C++, an OOP language, introduced the concept of classes, allowing for more structured and encapsulated code. The same functionality in C++ might be encapsulated in a class:
#include <iostream>
class Circle {
static const double PI;
public:
static double area(double radius) {
return PI * radius * radius;
}
};
const double Circle::PI = 3.14159;
int main() {
std::cout << "Area: " << Circle::area(5.0) << std::endl;
return 0;
}
In this example, Circle is a class with a static method area. The method is part of the Circle class but doesn’t require an instance of Circle to be called.
Static Utility Class Example in Java
We can introduce a static utility class that provides circle-related functionalities. This static utility class can offer a method to calculate the area of a circle, similar to the static approach used in C and C++, but within the Java ecosystem.
Let’s create a CircleUtils class that includes a static method to calculate the area of a circle. This utility class demonstrates how static methods can be used in Java, separate from the instance-based approach of the Circle class.
public class CircleUtils {
private static final double PI = 3.14159;
// Static method to calculate the area of a circle
public static double area(double radius) {
return PI * radius * radius;
}
}
public class Main {
public static void main(String[] args) {
double radius = 5.0;
double area = CircleUtils.area(radius);
System.out.println("Area of circle with radius " + radius + " is: " + area);
}
}
In this example, the CircleUtils class contains a static method area, which can be called without creating an instance of CircleUtils. This method is purely utility-based and does not maintain any state. It provides a clear and concise way to perform a common operation - calculating the area of a circle - using a static approach.
This approach is particularly useful when you need to perform operations that don’t require the data or state of an object, as is the case with calculating the area of a circle given its radius. It’s an excellent example of how static utility classes can coexist with OOP principles in Java, offering a simple and effective solution for certain types of tasks.
Java OOP Example
To complement the previous Java example with a static utility CircleUtils class, we can further emphasize the OOP paradigm in Java, promoting encapsulation and interaction through objects. Here’s how the circle area functionality would typically be represented:
public class Circle {
private static final double PI = 3.14159;
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double area() {
return PI * this.radius * this.radius;
}
public static void main(String[] args) {
Circle circle = new Circle(5.0);
System.out.println("Area: " + circle.area());
}
}
In this Java example, Circle is a class with a non-static method area and a constructor. An instance of Circle is created with a specific radius, and the area method calculates the area based on the radius of that instance. This represents a shift from using static methods to using instance methods, typical in OOP, where behavior is associated with object instances.
Current Trends in Programming Paradigms and Their Impact on Static Classes
In recent years, programming paradigms have continued to evolve, embracing concepts like functional programming, reactive programming, and microservices architecture. These paradigms often prioritize statelessness, immutability, and decentralization, which has further influenced the role of static classes.
For example, in functional programming languages like Haskell or Scala, the focus is on pure functions without side effects, a concept that doesn’t align well with traditional static classes that maintain state. Similarly, in microservices architectures, the emphasis on distributed, loosely coupled services challenges the centralized and often globally accessible nature of static classes.
Despite these shifts, static classes have not become obsolete. They have adapted to fit within these paradigms in certain contexts. In Java, for instance, static methods in utility classes remain a convenient and efficient way to perform common operations, such as string manipulation or mathematical calculations. However, their use is more judicious, with a greater focus on statelessness and immutability to align with modern software design principles.
Java’s introduction of default and static methods in interfaces from Java 8 onwards marked a significant evolution in interface design, allowing them to define behavior beyond mere method signatures. This change, however, does not signal a phase-out or complete replacement of static class methods. Static classes, especially utility classes with static methods, remain integral to Java programming for various reasons.
While the role of static classes has evolved and their use has become more nuanced in the face of new programming paradigms, they continue to be a valuable tool in the developer’s toolkit, albeit used with greater consideration for the overarching design principles of the application.
Static Classes in Modern Programming
In the modern programming landscape, static classes still hold a significant place, albeit with a more refined and targeted usage. The primary advantage of static classes lies in their simplicity and efficiency. Being able to access methods and variables directly through the class, without the need to instantiate an object, simplifies code and reduces overhead. This is particularly beneficial for utility functions, which are frequently accessed and do not require an object’s state.
Another advantage of static classes is their predictability and the ease of understanding they bring to certain parts of the code. For instance, in Java, classes like java.lang.Math and java.util.Arrays provide utility methods that are globally accessible and operate in a stateless manner. This global accessibility ensures that these utilities are consistently used throughout the application, promoting code reusability and maintainability.
Common Use Cases for Static Classes Today
Today, static classes are predominantly used in scenarios where the object-oriented paradigm does not offer additional value or where statelessness is preferred. Some common use cases include:
-
Utility Classes: These are perhaps the most common use case for static classes. They are used to group together related utility functions, like mathematical operations, string processing, or file handling. For example, the java.util.Collections class in Java provides a set of static methods for operating on collections, such as sorting and searching.
-
Constants: Static classes are an excellent place to define constants that are relevant across the application. For instance, a Constants class in a Java application might hold application-wide settings or configuration values.
-
Helper Functions: In scenarios where a set of functions provide auxiliary functionality (such as format conversions or validation checks) and do not need to maintain state, static helper classes can be effectively used.
Comparing Static and Non-Static Approaches in Software Design
The decision to use static or non-static classes often boils down to the specific requirements of the software design. Static classes are well-suited for situations where methods and data are independent of object state and need to be accessed globally. They offer simplicity and performance benefits in these scenarios. However, they can be limiting in terms of scalability and flexibility, as they cannot take advantage of polymorphism or dynamically change behavior at runtime.
Non-static classes, on the other hand, are the backbone of object-oriented programming. They allow for more flexible, scalable, and maintainable software design, especially in complex systems. Non-static classes enable the creation of objects that encapsulate state and behavior, making it easier to manage and modify the software over time. They also allow for inheritance and polymorphism, offering more dynamic and versatile solutions.
In modern software development, a balanced approach is often adopted. Developers choose static classes for utility and helper functions, where state management is not required, and prefer non-static classes for the core business logic and data representation, where the benefits of object-oriented principles are more pronounced. This hybrid approach leverages the strengths of both paradigms, leading to more efficient and maintainable codebases.
Utility Classes with Static Methods: A Closer Look
Utility classes with static methods are a fundamental part of software development, often serving as a toolkit for developers to perform common tasks more efficiently.
Definition and Purpose of Utility Classes in Programming
Utility classes are designed to provide a collection of static methods, usually related to a specific functionality or concept. These methods are typically stateless, meaning they don’t rely on or modify the internal state of an object. The primary purpose of utility classes is to group together useful, reusable functionalities that are not bound to a particular object but are rather generic in nature.
These classes enhance code readability, maintainability, and reusability by centralizing common methods in one place. They are particularly useful for operations that are widely needed across an application and don’t require object instantiation, such as mathematical calculations, string manipulation, or file operations.
The Structure and Characteristics of Utility Classes with Static Methods
A utility class is usually made final to prevent instantiation and inheritance, often contains a private constructor to prevent object creation, and consists exclusively of static methods and static variables (if any). Key characteristics include:
- Statelessness: Methods are designed to perform operations without depending on or altering the state of an object.
- Immutability: Since these classes are not meant to be instantiated, they are inherently immutable.
- Reusability: The methods are generic and can be reused across various parts of an application.
- Independence: These methods do not require interaction with instance data of a class.
Examples and Use Cases of Utility Classes in Various Programming Languages
Java Example: java.lang.Math
public final class Math {
private Math() {} // Private constructor
public static double sqrt(double a) {
return java.lang.StrictMath.sqrt(a); // Actual implementation may vary
}
// Other mathematical utility methods...
}
Use Case: Calculating the square root of a number without the need to create an instance of Math.
Python Example: os.path
In Python, modules can act as utility classes. The os.path module is a good example:
import os
path = "/user/docs/myfile.txt"
filename = os.path.basename(path) # Extracts the filename from a path
Use Case: Extracting the filename from a file path, using static methods provided by os.path.
C# Example: System.IO.Path
using System.IO;
class Program {
static void Main() {
string fullPath = @"C:\user\docs\report.txt";
string filename = Path.GetFileName(fullPath);
}
}
Use Case: Getting the filename from a full path string, using static methods of System.IO.Path.
In summary, utility classes with static methods are an essential part of programming, offering an organized, efficient way to access common functionalities across different programming languages. They are especially valuable in scenarios where operations are generic and can be separated from the concept of object state. These classes contribute significantly to writing cleaner, more maintainable, and efficient code.
Java’s Approach to Static Utility Classes
Java’s handling of static utility classes reflects its commitment to object-oriented programming principles while recognizing the need for efficient, accessible utility functions.
Overview of Java’s Implementation of Static Classes
In Java, static classes are often used to group utility methods that can be accessed independently of any object instance. A static utility class in Java typically has:
- A private constructor to prevent instantiation.
- All member variables and methods declared as static.
- Methods that are stateless and perform generic tasks.
These classes are central to Java’s design philosophy, providing a way to access common functionality in a way that’s both efficient and consistent with Java’s object-oriented nature.
Deep Dive into Java Utility Classes
Java provides several utility classes in its standard library, particularly within the java.util package. Key classes include:
java.util.Collections
The java.util.Collections class in Java serves as a comprehensive utility class, offering a wide range of static methods for tasks like sorting, synchronizing, and creating unmodifiable versions of collections. This class complements Java’s collection framework, enhancing the functionality and ease of manipulation of collection types within its object-oriented design.
java.util.List
While java.util.List itself is not a static utility class, it is frequently used with java.util.Collections, a static utility class that provides static utility methods for List objects. Notably, the Collections.sort(…) method, commonly used for sorting lists, internally redirects the call to List.sort(…).
java.util.Set
Similarly, java.util.Collections offers static methods like unmodifiableSet, which provides an unmodifiable view of a Set.
java.util.Map
java.util.Collections also offers methods like singletonMap, which creates an immutable map containing a single key-value pair.
java.util.Collection
In the broader context, java.util.Collections provides static utility methods that are applicable to all Collection types, including Lists, Sets, and others. Methods such as addAll, emptySet, synchronizedCollection are examples. These methods facilitate operations like adding multiple elements, creating empty or synchronized collections, applicable across different types of collections in a uniform manner.
Each of these interfaces (List, Set, Map, and Collection) demonstrates Java’s approach of combining specific, instance-based collection behaviors with the generalized, static utility methods provided by java.util.Collections. This blend offers a versatile toolkit for Java developers, enabling efficient manipulation of collections while adhering to object-oriented principles.
The Integration of Static and Default Methods in Java Interfaces
In addition to static utility classes, Java also allows static and default methods in interfaces. This feature, introduced in Java 8, enables interfaces to contain method implementations, not just abstract method declarations. This has broadened the scope of interfaces, allowing them to provide utility methods related to the interface’s purpose.
Java’s Approach to Static Utility Classes
The Integration of Static and Default Methods in Java Interfaces
Java’s approach to integrating static and default methods in interfaces represents a significant evolution in its object-oriented framework, particularly in the way it enhances the functionality and flexibility of collection interfaces.
Static Methods in Collection Interfaces
One of the key advancements in Java 8 was the introduction of static methods in interfaces. These methods are part of the interface but are not tied to an instance of the interface-implementing class. In the realm of collection interfaces, this feature has been leveraged to provide utility functions directly within the interface.
For instance, collection interfaces such as List, Set, and Map now include static methods like of(…), which offer a convenient way to create immutable collection instances. Here are some examples:
It’s noteworthy that even though [Collection].of(…) and alike provides a modern, concise way to create immutable lists in Java, under the hood, this method is still implemented using an internal utility class. This implementation detail reflects Java’s continued reliance on utility classes for efficient and optimized operations, even as it presents a more streamlined interface to developers through its collection APIs.
List.of(…)
Creates an immutable list from a given set of elements.
List<String> immutableList = List.of("Apple", "Banana", "Cherry");
Set.of(…)
Similar to List.of(…), this method creates an immutable set.
Set<Integer> immutableSet = Set.of(1, 2, 3);
Map.of(…)
Used to create an immutable map with key-value pairs.
Map<Integer, String> immutableMap = Map.of(1, "One", 2, "Two");
Pre JDK 9
Before JDK 9, when static methods like [Collection].of(…) and alike were not available, creating immutable lists in Java required a combination of using a concrete list implementation and then wrapping it with Collections.unmodifiableList(…). This approach was a bit more verbose compared to the concise syntax introduced in JDK 9 and later versions.
Here’s how you would create an immutable list using static utility classes in pre-JDK 9:
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
List<String> immutableList = Collections.unmodifiableList(list);
In this example, an ArrayList is first created and populated with elements. Then, Collections.unmodifiableList(…) is used to wrap the original list, resulting in an immutable view of the list. This was the standard way to create immutable collections before the introduction of convenient factory methods in JDK 8.
The Usage Pattern of Static Methods in Collection Interfaces
In the Java Collection Framework, the static methods provided in collection interfaces like List, Set, and Map act as facades to underlying static classes. These methods simplify the creation and manipulation of collections but internally leverage package-private utility classes to implement their functionality.
For instance, the static method <E> List<E> of(E e1) in the List interface provides a convenient way to create an immutable list. However, under the hood, this method typically delegates the actual creation of the list to a package-private utility class.
java.util.List.of(E) Source:
/**
* Returns an unmodifiable list containing one element.
*
* See <a href="#unmodifiable">Unmodifiable Lists</a> for details.
*
* @param <E> the {@code List}'s element type
* @param e1 the single element
* @return a {@code List} containing the specified element
* @throws NullPointerException if the element is {@code null}
*
* @since 9
*/
static <E> List<E> of(E e1) {
return new ImmutableCollections.List12<>(e1);
}
In the code snippet, the of(E e1) static method in the List<E> interface is shown to use the ImmutableCollections.List12<E> class to create an instance of an immutable list. This design pattern is common in the Java Collections Framework, where interface static methods provide a user-friendly API while the actual implementation details are abstracted away in specialized, often package-private classes. The remainder of the Collection interfaces java.util.Set, java.util.Map, etc.., follows the same facade pattern.
It’s important to recognize that in the Java Development Kit implementations, the newly introduced static and default methods in Collection interfaces primarily act as a facade. Internally, they utilize a package-private utility class, for example ImmutableCollections.List12<E>(), to handle a List.of(E) operation. This approach in Java elegantly masks the complexity of implementation details while providing a user-friendly API.
Default Methods in Interfaces
Alongside static methods, Java 8 also introduced default methods in interfaces. These methods have a default implementation and can be overridden in the implementing classes. This feature allows interfaces to evolve while maintaining backward compatibility with classes that implemented earlier versions of the interface.
In the context of collections, default methods have enabled interfaces to provide additional functionalities without forcing all implementing classes to redefine them. For example:
- The Iterable interface includes a default forEach method, allowing elements of any Iterable object (like a List or Set) to be iterated over using a consumer function.
- The List interface has been enhanced with default methods like replaceAll and sort, enabling in-place modification of lists.
The integration of static and default methods into Java interfaces represents a thoughtful blending of static utility concepts with the dynamism and flexibility of object-oriented design. It enables interfaces to serve not just as contracts for implementing classes but also as repositories for shared utility functionalities and evolving behaviors. This approach reflects Java’s ongoing adaptation to modern programming paradigms, balancing the legacy of its object-oriented roots with the innovative features of functional programming.
Examples of Static Utility Classes in Java and Their Applications
java.lang.Math
double result = Math.sqrt(25); // Calculates the square root
Use Case: Performing a mathematical operation without the need for instantiating any object.
java.util.Arrays
int[] array = {3, 5, 1, 4, 2};
Arrays.sort(array); // Sorts the array
Use Case: Sorting an array using a static method.
java.util.Collections
List<String> list = new ArrayList<>();
Collections.addAll(list, "Apple", "Banana", "Cherry");
Collections.sort(list); // Sorts the list
Use Case: Manipulating collections like sorting or adding all elements at once.
Java’s approach to static utility classes and methods demonstrates a balance between the principles of object-oriented programming and the practical need for accessible, efficient utility functions. These classes and methods provide essential functionalities across Java applications, exemplifying how static and object-oriented features can coexist effectively in a programming language.
Interface Default and Static Methods in Java
The introduction of default and static methods in Java interfaces, particularly from Java 8 onwards, marked a significant evolution in Java’s capability, allowing interfaces to be more than just a contract for implementing classes.
Introduction to Default and Static Methods in Java Interfaces
Prior to Java 8, interfaces in Java could only declare methods, not implement them. This changed with two major additions:
-
Default Methods: These are methods within an interface that provide a default implementation. They allow developers to add new methods to interfaces without breaking the existing implementation of these interfaces.
-
Static Methods: These are methods within an interface that are static, meaning they can be called independently of any object of the class that implements the interface. This feature allows interfaces to provide utility-like methods.
Evolution of Java Interfaces with Static Methods
Static methods in interfaces brought a utility-class-like capability directly into interfaces. Before Java 8, any utility method related to an interface had to be placed in a separate utility class (like java.util.Collections). With static methods, these utility functionalities can now be part of the interface itself, making the interface a one-stop location for both the contract and related utilities.
Examples and Practical Applications
Default Methods Example
Consider an interface Vehicle that has been around for some time. With Java 8, you can add a new method with a default implementation without breaking existing code:
public interface Vehicle {
void start();
default void turnOnRadio() {
System.out.println("Radio turned on!");
}
}
public class Car implements Vehicle {
public void start() {
System.out.println("Car started");
}
}
// In use:
Car car = new Car();
car.start(); // Output: Car started
car.turnOnRadio(); // Output: Radio turned on! (uses default method)
Static Methods Example
Static methods in interfaces can be useful for utility purposes. For example, a Polygon interface can have a static method to create an instance:
public interface Polygon {
int getNumberOfSides();
static Polygon createTriangle() {
return () -> 3; // A simple implementation returning a triangle
}
}
// In use:
Polygon triangle = Polygon.createTriangle();
System.out.println(triangle.getNumberOfSides()); // Output: 3
Default and static methods in Java interfaces have added a new dimension to interface design in Java. Default methods provide flexibility in evolving interfaces without breaking existing implementations, while static methods offer a way to include utility functions directly within interfaces. This integration enhances the expressiveness and functionality of interfaces in Java, aligning them with modern programming paradigms.
Static Classes: A Comparative Analysis
The use of static classes in software development has been a subject of considerable debate, with arguments for and against their use in various contexts.
Pros and Cons of Using Static Classes in Modern Software Development
Pros
- Simplicity and Accessibility: Static classes offer a straightforward way to group related utility functions, making them globally accessible without the need for object instantiation.
- Performance Efficiency: Since there’s no need to create an instance, static methods can be more efficient for frequently used utility functions.
- Statelessness: Static methods typically don’t maintain internal state, reducing side effects and enhancing predictability.
Cons
- Testing Challenges: Static methods can be challenging to mock during unit testing, which can lead to difficulties in testing classes that depend on them.
- Tight Coupling: Excessive use of static methods can lead to tightly coupled code, making it harder to modify, extend and test.
- Inflexibility: Unlike instance methods, static methods are not overrideable, which can limit flexibility and reuse in certain design scenarios.
Static classes remain a valuable tool in the developer’s toolkit but are increasingly being used with more consideration for their impact on code quality, flexibility, and maintainability. The trend is towards a judicious use of static classes, balancing their benefits with the principles of modern software architecture and design.
The performance difference between static importing static class methods and implementing an interface with default or static methods is typically negligible in most practical scenarios. Both approaches serve different purposes and have their own advantages and use cases.
Static Importing Static Class Methods
- Static importing is used to bring static methods and fields of a class into the current class’s namespace, allowing you to use them without referencing the class name explicitly.
- Static methods from imported classes are invoked directly, and there is very little overhead associated with this.
Implementing an Interface with Default or Static Methods
- Implementing an interface with default or static methods allows you to provide default implementations for methods in an interface.
- When you call default or static interface methods, they are invoked just like regular method calls, and there is minimal performance overhead.
In terms of performance, both approaches are efficient because the overhead of method invocation is generally very low. Performance concerns should not be the primary factor in deciding between these two approaches; instead, focus on the design and organization of your code to make it more maintainable and readable.
The Future of Static Classes
The future of static classes in programming, amidst evolving technologies and paradigms, suggests a shift towards more selective and strategic use. As development practices advance, static classes are likely to be employed for specific utility purposes, complementing the flexibility offered by object-oriented and functional programming.
The integration with new programming features may further refine their usage. Additionally, the growing focus on cloud computing, microservices, and functional programming could influence a reduced reliance on static classes, favoring more dynamic and decoupled designs.
In essence, while static classes will remain a part of the programming landscape, their application is expected to be more targeted and nuanced, aligning with the trends towards testability, maintainability, and adaptability in software development.
Java • Mastering New Stream Collector Methods
Post Date: 02 Jan 2024
Stream processing in Java has revolutionized how we handle data, offering a functional approach to manipulate collections. With the release of new versions, Java continues to enhance this capability, introducing more intuitive and concise methods to collect and transform data streams.
Java • Dynamic Proxy vs CGLIB
Post Date: 28 Dec 2023
The comparison between Java Dynamic Proxy and CGLIB represents a critical discussion in the realm of Java programming. In this article, we explore the distinct features, advantages, and use cases of Java Dynamic Proxy and CGLIB, offering insights for developers to make informed choices in their projects.
Embed from Getty Images
Java Dynamic Proxy, a part of the Java Reflection API, and CGLIB, a powerful, high-performance code generation library, each bring unique capabilities to the table.
Java • Beginners Guide To Reflection
Post Date: 28 Dec 2023
Java Reflection is a pivotal feature in Java programming, offering dynamic class manipulation. This guide introduces Java Reflection to beginners, illustrating its significance for Java developers. Reflection allows for runtime interactions with classes, enabling tasks like accessing private fields and methods, and creating objects dynamically.
Intro To Java Dynamic Proxies
Post Date: 27 Dec 2023
Java dynamic proxies represent a powerful and often underutilized feature in the Java programming language. At its core, a Java dynamic proxy is a mechanism that allows developers to create a proxy instance for interfaces at runtime. This is achieved through Java’s built-in reflection capabilities. Dynamic proxies are primarily used for intercepting method calls, enabling developers to add additional processing around the actual method invocation.
Java • Intro To CGLIB Proxies
Post Date: 27 Dec 2023
In this introductory article, we delve into the world of CGLIB Proxies, a powerful tool for enhancing the functionality of Java applications. We explore how CGLIB, as a bytecode generation library, offers dynamic proxy capabilities, essential for developers looking to create robust and flexible software.
Mastering Java Parallel Streams: Enhancing Performance in Modern Applications
Post Date: 24 Dec 2023
Java’s Evolution to Parallel Streams: Java, an ever-evolving and versatile programming language, has made significant strides in adapting to the dynamic landscape of modern application development. A landmark in this journey was the introduction of parallel streams with Java 8, a feature that fundamentally transformed how developers optimize performance and enhance efficiency in their applications.
Java • Guide to Stream Concatenation
Post Date: 24 Dec 2023
Java, a versatile and widely-used programming language, offers robust features for data handling, one of which is stream concatenation in its API. Stream concatenation allows developers to combine multiple data streams efficiently, enhancing data processing capabilities in Java applications. This article delves into the nuances of stream concatenation, providing insights and best practices for Java developers looking to optimize data handling in their applications.
Java • ThreadLocal Alternatives
Post Date: 22 Dec 2023
In this article, we delve into the realm of Java concurrency, focusing on ThreadLocal and its alternatives. ThreadLocal is a fundamental tool in Java for managing thread-scoped data, but it’s not without its drawbacks. We’ll explore the challenges associated with ThreadLocal, shedding light on why developers often seek alternatives. The article will also introduce ScopedValue, a less familiar but significant option, and compare it with ThreadLocal.
Java • Intro to InheritableThreadLocal
Post Date: 22 Dec 2023
In the realm of Java programming, InheritableThreadLocal stands out as a pivotal yet frequently overlooked component, especially in the domain of sophisticated multithreading. This distinctive feature in Java’s concurrency toolkit allows data to be passed seamlessly from a parent thread to its child threads, ensuring a level of continuity and state management that is crucial in complex applications.
Java • Try With Resources Practical Example
Post Date: 21 Dec 2023
Java’s introduction of the try-with-resources statement revolutionized resource management, simplifying code and enhancing reliability. This feature, integral to Java’s exception handling mechanism, automatically manages resources like files and sockets, ensuring they are closed properly after operations, thus preventing resource leaks. Our discussion will delve into a practical example to understand how try-with-resources works and its benefits over traditional resource management techniques.
Java • ThreadLocal vs Thread
Post Date: 21 Dec 2023
Java, as a versatile and powerful programming language, offers various mechanisms to handle multithreading and concurrency. Two such concepts, Thread and ThreadLocal, are pivotal in Java’s approach to multi-threaded programming. Understanding the distinction between these two, as well as their respective advantages and limitations, is crucial for any Java developer aiming to write efficient and robust multi-threaded applications.
Java • ThreadLocal Usecase In Servlet Filters
Post Date: 19 Dec 2023
ThreadLocal in Java serves as a powerful mechanism for ensuring thread safety and managing data that is specific to individual threads, especially in multi-threaded environments like web servers. This article delves into the application of ThreadLocal in the context of Servlet Filters, an integral part of Java web applications. We explore how ThreadLocal can be strategically used to enhance performance, maintain clean code, and ensure thread safety in Servlet Filters, making your Java web applications more robust and efficient.
Java • Understanding the Dangers of ThreadLocal
Post Date: 19 Dec 2023
In this article, we delve into the intricate world of Java programming, focusing on a specialized feature: ThreadLocal. Known for its ability to store data specific to a particular thread, ThreadLocal plays a crucial role in Java’s multi-threading capabilities. However, it’s not without its pitfalls. This exploration aims to unravel the complexities and potential dangers associated with ThreadLocal, providing insights for both seasoned and budding Java developers.
Java • ThreadLocal Best Practices
Post Date: 19 Dec 2023
Java’s ThreadLocal is a powerful yet intricate component in concurrent programming, offering unique challenges and opportunities for developers. This article delves into the best practices for using ThreadLocal in Java, ensuring optimal performance and maintainability. By understanding its proper usage, developers can harness the full potential of ThreadLocal to manage data that is thread-specific, thereby enhancing application efficiency and robustness in multi-threaded environments.
Java • Logback Mapped Diagnostic Context (MDC) in Action
Post Date: 18 Dec 2023
Java’s Logback framework offers a robust and flexible logging system, pivotal for any software development project. Among its features, the Mapped Diagnostic Context (MDC) stands out for its utility in providing contextual information in log messages.
Java • Logback Propagating MDC To Child Thread
Post Date: 16 Dec 2023
Java’s Logback framework stands as a robust logging tool in Java applications, known for its enhanced flexibility and configurability. A pivotal feature of Logback is the Mapped Diagnostic Context (MDC), instrumental in enriching log messages with context-specific information. However, developers often encounter the challenge of propagating MDC data to child threads, a key step in maintaining contextual continuity in multi-threaded environments.
Java • Logback MDC In Thread Pools
Post Date: 16 Dec 2023
Java Logback, a versatile logging framework, is essential for developers seeking efficient debugging and monitoring solutions. This article dives into the nuances of managing the Mapped Diagnostic Context (MDC) within a thread pool environment, a scenario common in Java applications. We’ll explore how Logback’s sophisticated features can be leveraged to handle MDC data safely and efficiently, ensuring thread safety and data integrity.
Spring • Intro To Aspect-Oriented Programming
Post Date: 15 Dec 2023
Aspect-Oriented Programming (AOP) is an innovative programming paradigm that addresses concerns that cut across multiple classes in application development, such as logging, security, or transaction management. Spring AOP, a key component of the widely-used Spring Framework, provides an elegant solution to handle these cross-cutting concerns efficiently and in a modular way.
Java • Understanding Role Of Classloader
Post Date: 14 Dec 2023
In this article, we delve into the intricacies of Java’s Classloader, a fundamental component of the Java Runtime Environment (JRE) that plays a crucial role in how Java applications run. We’ll explore the concept of Classloader, its functionality, and its significance in Java programming. By demystifying this complex element, the article aims to provide readers with a clear understanding of how Java classes are loaded and managed, enhancing their grasp of Java’s operational mechanisms.
What Is a Java Bytecode
Post Date: 12 Dec 2023
Java bytecode is a crucial element in the world of Java programming, serving as the intermediate representation of Java code that is executed by the Java Virtual Machine (JVM). This article aims to demystify Java bytecode, breaking down its structure, purpose, and functionality.
Java • How To Get Package Name
Post Date: 12 Dec 2023
Java, a robust and widely-used programming language, offers various ways to interact with its core components, such as packages and classes. Understanding how to retrieve package names in Java is crucial for developers, especially when dealing with large, complex projects.
Java • Pitfalls of Returning Null
Post Date: 10 Dec 2023
In the realm of Java programming, the use of null has been a topic of extensive discussion and analysis. This article delves into the nuances of returning null in Java, exploring its implications, best practices, and viable alternatives.
Initially, we will examine the concept of null in Java, its usage, and why it often becomes a source of debate among developers.
Java Streams • filter() & map() Beyond Basics
Post Date: 09 Dec 2023
Delving into the advanced aspects of Java Streams, this article ventures beyond the elementary use of filter() and map() functions. Aimed at developers who have a grasp on the basics, this piece aims to elevate your understanding to a more sophisticated level.
Java Optional • Common Mistakes and Misconceptions of map() & flatMap()
Post Date: 09 Dec 2023
Java’s Optional class, introduced in Java 8, is a pivotal tool for handling nulls effectively in Java applications. However, its map() and flatMap() methods often become sources of confusion and mistakes for many developers. This article dives into the intricacies of these methods, uncovering common misconceptions and errors.
Java Optional • map() vs flatMap()
Post Date: 08 Dec 2023
In this article, we delve into the intricate world of Java’s Optional class, focusing on two pivotal methods: map() and flatMap(). We’ll explore how these functions enhance code readability and error handling in Java, offering a nuanced understanding of their usage and benefits. The comparison between map() and flatMap() will illuminate their roles in functional programming, elucidating when and why to use each method effectively.
Java Stream • findFirst() and findAny() In Action
Post Date: 07 Dec 2023
In the realm of Java programming, stream operations offer powerful tools for processing sequences of elements. Among these, the findFirst() and findAny() methods are pivotal in retrieving elements from a stream. This article delves into the nuances of these methods, explicating their functionalities, differences, and appropriate use cases. Understanding these methods is crucial for Java developers looking to harness the full potential of stream processing.
Java • int vs long
Post Date: 07 Dec 2023
In Java programming, understanding data types is crucial for efficient and error-free coding. Two fundamental data types often encountered are int and long. This article delves into their differences, use cases, and how they impact Java applications. By comprehending the nuances between these types, developers can make informed decisions, optimizing their code for performance and precision.
Java • AtomicReference Expert Guide
Post Date: 07 Dec 2023
AtomicReference in Java is an intriguing feature that enhances the thread-safety of your applications. This guide dives into the intricacies of AtomicReference, explaining its functionality, benefits, and practical usage in Java development. We’ll explore its comparison with similar atomic classes and provide insights on when and how to effectively implement it in your projects.
Java • Custom Annotations In Action
Post Date: 06 Dec 2023
In the dynamic landscape of Java programming, custom annotations have become a pivotal tool, revolutionizing code development and maintenance. As specialized metadata, custom annotations in Java empower developers to infuse additional information into their code, enhancing readability, maintainability, and functionality. They simplify complex tasks like serialization and data validation, and improve communication in collaborative coding environments.
Functional Programming with Java
Post Date: 03 Dec 2023
Functional Programming (FP) in Java marks a significant shift towards a more efficient and clean coding paradigm, integrating core principles like immutability, pure functions, and higher-order functions into its traditional object-oriented framework. This article delves into the pivotal role of lambda expressions and the Stream API in enhancing code readability and performance.
Java vs. C#
Post Date: 29 Nov 2023
In the dynamic and ever-evolving world of software development, Java and C# stand as two titans, each with its own unique strengths, philosophies, and ecosystems. This article delves into an in-depth comparison of Java and C#, exploring their historical context, language features, performance metrics, cross-platform capabilities, and much more.
Java • Mockito vs EasyMock
Post Date: 26 Nov 2023
Java, a widely-used programming language, has evolved significantly over the years, especially in the realm of testing. In this digital era, where software development is fast-paced and highly iterative, the importance of efficient and reliable testing frameworks cannot be overstated. Among the various tools and libraries available for Java developers, Mockito and EasyMock stand out as popular choices for unit testing.
Java • Single Responsibility Principle
Post Date: 23 Nov 2023
The Single Responsibility Principle (SRP), a fundamental concept within the SOLID principles, is crucial in Java programming. It dictates that each class should have only one reason to change, focusing on a single functionality or concern. This approach is particularly effective in Java, known for its robust object-oriented features, where SRP enhances maintainability, readability, and scalability of applications.
Java • Multiple Inheritance Using Interface
Post Date: 22 Nov 2023
Amongst the many facets of object-oriented programming, the concept of inheritance is fundamental. Multiple inheritance, a feature where a class can inherit from more than one superclass, can be particularly powerful but also complex. Java, however, does not support multiple inheritance directly in the way languages like C++ do. Instead, it offers a robust alternative through interfaces.
Java • Interfaces Are Replacing Abstract Classes
Post Date: 22 Nov 2023
The Java programming language, renowned for its robust structure and versatile capabilities, has witnessed a notable evolution in its fundamental components over the years. Among these, the role and functionality of interfaces and abstract classes have undergone significant changes, particularly with the introduction of new features in Java 8.
Java • Decoupling Arbitrary Objects Through Composition
Post Date: 22 Nov 2023
In the dynamic landscape of software development, the concept of object decoupling plays a pivotal role in crafting efficient, maintainable, and scalable applications. At its core, object decoupling refers to the design approach where components of a program are separated in such a manner that they are independent, yet functionally complete. This separation ensures that changes in one part of the system minimally impact other parts, facilitating easier updates, debugging, and enhancement.
Java Primitives & Primitive Wrappers
Post Date: 16 Nov 2023
Java, a robust and widely-used programming language, stands out for its efficient handling of data types. Central to its functionality are the Java primitives and their corresponding wrapper classes. This article delves into the essence of Java primitives, their types, and the distinction between primitive and non-primitive data types, including examples to illustrate these concepts.
Java • Primitive int vs Integer Best Practices
Post Date: 07 Nov 2023
In Java, one of the foundational decisions developers must make pertains to choosing between primitive types and their corresponding wrapper classes, such as int and Integer. Both have their place in Java applications, and understanding their differences is paramount for writing efficient and effective code.
Java • Harnessing Static and Default Methods in Interfaces
Post Date: 06 Nov 2023
The arrival of static and default methods in Java 8 marked a significant shift in interface capabilities, expanding their functionality and versatility in Java’s object-oriented ecosystem. This article explores the nuances of these features and their impacts on Java programming, simplifying complex concepts and illustrating their practical applications in modern software development.
Java Modern Collection Utilities
Post Date: 06 Nov 2023
Java’s evolution has always been about simplifying complexity and enhancing efficiency. The collection utilities have undergone significant improvements since JDK 8, transitioning from the Collections utility class to the intuitive List.of(), Map.of(), and Set.of() methods.
Java • AssertJ vs Hamcrest Assertion Frameworks
Post Date: 27 Oct 2023
When working with testing frameworks like JUnit or TestNG, selecting the right assertion framework can significantly enhance the readability of your test code and improve the overall quality of your tests. Two of the most popular Java assertion frameworks are AssertJ and Hamcrest.
Java • Unit Testing Best Practices
Post Date: 26 Oct 2023
Unit testing is a fundamental aspect of software development, ensuring that each individual unit of source code is thoroughly examined and validated for correctness. With Java being one of the most widely used programming languages, it is crucial to adhere to the best practices for unit testing in Java to maintain the integrity and performance of the software.
Logback for Beginners
Post Date: 19 Oct 2023
Logback, a Java-based logging framework within the SLF4J (Simple Logging Facade for Java) ecosystem, is the preferred choice in the Java community, serving as an enhanced successor to the popular Log4j project. It not only carries forward the legacy of Log4j but also brings to the table a quicker implementation, more comprehensive configuration options, and enhanced flexibility for archiving old log files.
Java • Modern Looping And Filtering with Stream API
Post Date: 19 Oct 2023
Java has constantly evolved since its inception, presenting developers with numerous tools and methods to make coding more efficient and readable. Among these are modern techniques for looping and filtering data.
Java • Converting Strings To List
Post Date: 19 Oct 2023
When it comes to working with Java, converting strings into lists is a common and essential operation that can significantly enhance your data processing capabilities. Whether you’re a seasoned programmer or just starting, mastering this technique will prove to be invaluable in your coding endeavors.
Java var Best Practices
Post Date: 18 Oct 2023
Java, with each release and update, continually evolves to simplify the developer’s journey while preserving its core tenets of readability and robustness. One of the notable introductions in Java 10 was the var keyword. As with most new features, it sparked debates and questions regarding its efficacy and best practices.
URI vs URL in Java
Post Date: 16 Oct 2023
In the realm of Java and web development, the terms URL and URI often emerge in discussions, leaving some in a quagmire of confusion. This article aims to elucidate the disparities between the two, elucidating their syntax, utilization in Java, and the nuances that set them apart.
Java vs JavaScript • Which Is In More Demand?
Post Date: 02 Oct 2023
Java and JavaScript, despite their similar names, serve distinct purposes within the realm of software development. As both languages continue to evolve and find niches in the modern tech landscape, it’s crucial to understand their differences and their respective market demands.
Java Cloning Strategies
Post Date: 23 Jun 2023
Object copying is a fundamental aspect of Java programming, finding relevance and utility in diverse contexts. Whether it’s creating independent copies of objects, maintaining object state, or avoiding unintended side effects, understanding efficient and reliable cloning strategies is essential.
Java Comprehensive Guide
Post Date: 17 May 2023
Java is a versatile programming language that has gained widespread popularity for its platform independence and robustness. In this comprehensive guide, we will delve into the various aspects of Java programming, covering essential concepts, tools, and best practices.
Java • Converting Strings To Map
Post Date: 03 May 2023
This article discusses converting a string of key-value pairs that are delimited by a specific character, known as a delimiter, into a Map in Java.
Maven vs Gradle
Post Date: 01 May 2023
Maven and Gradle are two of the most popular build automation tools for Java-based projects. Both tools are designed to simplify the build process, manage dependencies, and facilitate project organization.
Java 19 Virtual Threads
Post Date: 04 Apr 2023
In this article, we will provide an overview of virtual threads in Java and their use in concurrent programming. We will define what virtual threads are and how they differ from normal threads. Additionally, we will discuss the benefits of virtual threads over traditional concurrency approaches and provide code examples to illustrate the differences between the two.
Decoupling Domain Objects: Simplifying System Architecture
Post Date: 31 Mar 2023
When you design an object-oriented system from top to bottom, sometimes the objects that represent the “domain” (what the system is about) don’t match the objects that represent the “entities” (what the system stores). To solve this problem, you can use a technique called “decoupling” to separate the layers of objects.
Java Final Modifier
Post Date: 27 Mar 2023
In Java, the final keyword (also known as a modifier) is used to mark a variable, method, or class as immutable, meaning its value or behavior cannot be modified once it has been initialized.
Java Records
Post Date: 14 Mar 2023
A Java record is a new feature introduced in Java 14 that allows developers to create a class that is primarily used to store data. A record is essentially a concise way to define a class that consists mainly of state (fields) and accessors (getters).
Java 17 Features
Post Date: 14 Mar 2023
JDK 17, introduces several new features and improvements, including enhanced random number generators, new encoding-specific methods for the String class, and default classes for Java ciphers. It also removes the experimental AOT and JIT compilers, and introduces support for Sealed Classes and Records. These changes provide developers with more flexibility and control, making it easier to write efficient and secure Java applications.
Java Optional - Why Developers Prefer Optional Values
Post Date: 12 May 2019
This article discusses the use of Java Optional to introduce optional values instead of null. We will deep dive into understanding why developers prefer the Optional class to clearly communicate an optional value as opposed to a vague null representation of a variable.
Java • Int to String Conversion Guide
Post Date: 11 May 2019
In Java, often times the ability to return a string representing the specified integer is a common task. This article illustrates several mechanisms to convert int to a string in Java. In the opposite scenario, the means to resolve an integer representing the value of the specified String. The returned value is an Integer object that is the equivalent integer value of the argument string.
Java • Double to String Conversion | Beginner's Guide
Post Date: 11 May 2019
Converting double to a String value in Java has been a typical task to do for software development. This article discusses the various ways on how to convert a double to a string in Java. While there are advantages in representing a double to its String object representation, the opposite task of converting a String object to a double can also be addressed. This document examines the reasons why conversions of double in Java are beneficial for beginners who are learning to develop in java.
Setting Java Compiler Version in Maven
Post Date: 27 Aug 2018
This document demonstrates ways to set the java compiler version in maven via the maven.compiler.target property and the maven-compiler-plugin configuration section.
Getting Started With Java
Post Date: 15 Aug 2018
The following page will illustrate how to get started with the Java Programming
Language. In addition, this document provides an overview of how to install
java and the environment variables you will need to set. A hands-on approach
illustrates how to compile and run your first Hello World java code.
Getting Started With Gradle
Post Date: 14 Aug 2018
The following page will be an excellent guide with getting started with the gradle build system in your
Java™ projects. Use this guide as a reference when using Gradle as a build system for the very first time.